Multiple custom content scroller With jquery
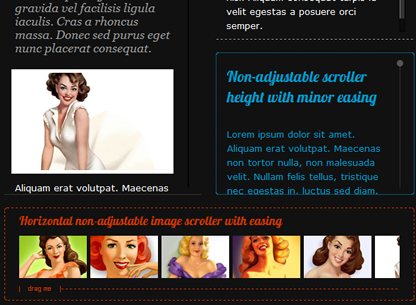
Custom scrollbar plugin utilizing jquery UI that’s fully customizable with CSS. It features vertical/horizontal scrolling, mouse-wheel support (via Brandon Aaron jquery mouse-wheel plugin), scroll easing and adjustable scrollbar height/width.
In order to implement the plugin, you first need to insert inside the head tag of your document the jquery.min.js and jquery-ui.min.js (both loaded from Google), the jquery.easing.1.3.js (the plugin that handles animation easing), jquery.mousewheel.min.js (to support mouse-wheel functionality) and the jquery.mCustomScrollbar.css which is the file where you can style your content and scrollbars.
<link href="jquery.mCustomScrollbar.css" rel="stylesheet" type="text/css" /> <script src="http://ajax.googleapis.com/ajax/libs/jquery/1.4/jquery.min.js" type="text/javascript"></script> <script src="http://ajax.googleapis.com/ajax/libs/jqueryui/1.8/jquery-ui.min.js" type="text/javascript"></script> <script src="jquery.easing.1.3.js" type="text/javascript"></script> <script src="jquery.mousewheel.min.js" type="text/javascript"></script>
Next you’ll need to insert the markup of the content blocks you want to have custom scrollbars inside the body tag. The structure of the markup is exactly the same for every content block except the first div which should have a unique id (in this example: mcs_container).
<div id="mcs_container"> <div class="customScrollBox"> <div class="container"> <div class="content"> <p>Your long content goes here...</p></div> </div> <div class="dragger_container"></div> </div> </div>
Keep the markup structure exactly as shown above, placing your content inside the div that has the class name content. In order to have multiple content blocks with custom scrollbars on a single document, you use the exact same markup with a unique id for each block (e.g. mcs_container, mcs2_container, mcs3_container etc.).
To add the additional scrollbar buttons, you need to insert two additional anchor tags inside mcs_container div, with class names scrollUpBtn and scrollDownBtn:
<div id="mcs_container"> <div class="customScrollBox"> <div class="container"> <div class="content"> <p>Your long content goes here...</p></div> </div> <div class="dragger_container"></div> </div> <!-- scroll buttons --> <a class="scrollUpBtn" href="#"></a> <a class="scrollDownBtn" href="#"></a> </div> </div></div>
An extra feature suggested and provided by isHristov is to add a few lines of CSS right after your content markup, in order to help users that have javascript disabled scroll the content:
<noscript> <style type="text/css"> #mcs_container .customScrollBox{overflow:auto;} #mcs_container .dragger_container{display:none;} </style> </noscript>
The final step is to include the actual custom scrollbar plugin (jquery.mCustomScrollbar.js) and the function that calls and configures the scrollbar(s) at the end of your document, just before the closing body tag.
<script> $(window).load(function() { $("#mcs_container").mCustomScrollbar("vertical",400,"easeOutCirc",1.05,"auto","yes","yes",10); }); </script> <script src="jquery.mCustomScrollbar.js" type="text/javascript"></script>
You can configure each content block scrollbar by setting the parameters of the function call:
- The scroll type (“vertical” or “horizontal”)
- The scroll easing amount (e.g. 400 for normal easing, 0 for no easing etc.)
- The scroll easing type
- The extra bottom scrolling space (applies to vertical scroll type only)
- Set the scrollbar height/width adjustment (“auto” for adjustable scrollbar height/width analogous to content length or “fixed” for fixed dimensions)
- Set mouse-wheel support (“yes” or “no”)
- Scrolling via buttons support (“yes” or “no”)
- Buttons scrolling speed (an integer between 1 and 20, with 1 indicating the slowest scroll speed)
To set multiple content blocks with custom scrollbars on a single page, give them a unique id (keeping the exact markup) and add a function call for each one. For example:
<script> $(window).load(function() { $("#mcs_container").mCustomScrollbar("vertical",400,"easeOutCirc",1.05,"auto","yes","yes",10); $("#mcs2_container").mCustomScrollbar("vertical",0,"easeOutCirc",1.05,"fixed","yes","no",0); $("#mcs3_container").mCustomScrollbar("vertical",900,"easeOutCirc",1.25,"auto","no","no",0); }); </script>
Feel free to use it wherever and however you like. Enjoy
You might also like
Tags
accordion accordion menu animation navigation animation navigation menu carousel checkbox inputs css3 css3 menu css3 navigation date picker dialog drag drop drop down menu drop down navigation menu elastic navigation form form validation gallery glide navigation horizontal navigation menu hover effect image gallery image hover image lightbox image scroller image slideshow multi-level navigation menus rating select dependent select list slide image slider menu stylish form table tabs text effect text scroller tooltips tree menu vertical navigation menu