jQuery Frequently Asked Questions (FAQ)
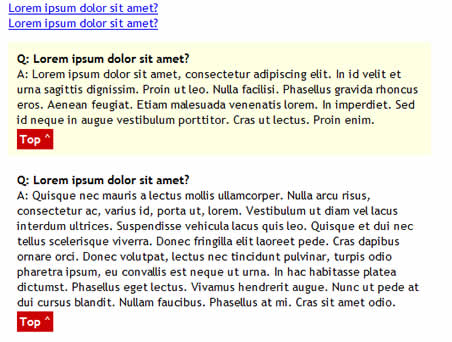
This time around we'll to create a FAQ page at work for a new website we are launching.
Creating the FAQ
Obviously, you'll need a page with FAQ's on it. I personally chose to put everything in UL's, but you can put it in any list or div's. The only thing is it will greatly help if you have a wrapper so jQuery doens't have to search the entire page.
Each FAQ should have an anchor tag on it so the links at the top know where to point. This will look like:
<li><a name="1a"></a>Q: Lorem ipsum dolor sit amet?A: Lorem ipsum dolor sit amet.</li>
The anchor doesn't need to encompass any text, it's just a flag to say "Here's this!" and the name is just saying "Here's 1a". Make sure each name is different since we don't want the links up top to point to every question. Next you'll want to put links to your FAQ's at the top so people can access them easily. This will look like:
<ul> <li><a href="#1a">Lorem ipsum dolor sit amet?</a></li> </ul>
Again, I using UL's but you can use whatever you want. Also make sure there is a hash (#) and just a hash (no long url's) and that the link after the hash ('1a') matches what the name attribute of the question's anchor was.
As or right now, you have what the majority of FAQ's are. But that's not why you came here. So now for the jQuery. In the head of the page, put:
<style type="text/css"> .highlight { background: #ffffe1; } </style>
This will be the background color for the question the user clicked to highlight it for them.
<script> $(document).ready(function () { $(".top_links > li > a").click(function () { $(".faq > li").removeClass('highlight'); var str = $(this).attr("href"); str = "a[name='" + str.substring(1) + "']"; $(str).parent().addClass('highlight'); }); }); </script>
What this is is, jQuery's start "(document).ready". Then jQuery adds an onClick listener to all the li elements within the element(s) with the class top_links (which will be the top links). As I said before, it helps to have a wrapper. So if you did div's, you would put the wrapper's class instead of top_links then "> *". So all together ".wrapper > *". This says apply the listenter to all elements within the wrapper. Next, it removes the class highlight from any li's within the .faq element (these will be your actual questions) so multiple questions won't be highlighted. Then it takes the link that was clicked, dissect's the everything after the hash adds the correct formatting for jQuery and adds the class highlight to the parent class of the anchor.
You're done! Your page should have more than one question and some styling, but here is the basics of the page all put together
<html> <title>jQuery Frequently Asked Questions (FAQ) - Demo</title> <script src="http://ajax.googleapis.com/ajax/libs/jquery/1.2.6/jquery.min.js" type="text/javascript"></script> <script type="text/javascript"> $(document).ready(function () { $(".top_links > li > a").click(function () { $(".faq > li").removeClass('highlight'); var str = $(this).attr("href"); str = "a[name='" + str.substring(1) + "']"; $(str).parent().addClass('highlight'); }); }); </script> <style> .highlight { background: #ffffe1; } </style> <body> <ul class="faq"> <ul class="top_links"> <li><a href="#1a">Lorem ipsum dolor sit amet?</a></li> </ul> <li><a name="1a"></a>Q: Lorem ipsum dolor sit amet?A: Lorem ipsum dolor sit amet, consectetur adipiscing elit. In id velit et urna sagittis dignissim. Proin ut leo. Nulla facilisi. Phasellus gravida rhoncus eros. Aenean feugiat. Etiam malesuada venenatis lorem. In imperdiet. Sed id neque in augue vestibulum porttitor. Cras ut lectus. Proin enim.</li> </ul> </body> </html>
But wait! As I said earlier, if you want to add LocalScroll to your FAQ, visit his site and download both LocalScroll and ScrollTo. Next add them to the head like this:
<script src="jquery.localscroll.js" type="text/javascript"></script> <script src="jquery.scrollTo.js" type="text/javascript"></script>
Then initialize it within the (document).ready where ever you like. You're head script tag should look like:
<script> $(document).ready(function () { $.localScroll(); $(".top_links > li > a").click(function () { $(".faq > li").removeClass('highlight'); var str = $(this).attr("href"); str = "a[name='" + str.substring(1) + "']"; $(str).parent().addClass('highlight'); }); }); </script>
You might also like
Tags
accordion accordion menu animation navigation animation navigation menu carousel checkbox inputs css3 css3 menu css3 navigation date picker dialog drag drop drop down menu drop down navigation menu elastic navigation form form validation gallery glide navigation horizontal navigation menu hover effect image gallery image hover image lightbox image scroller image slideshow multi-level navigation menus rating select dependent select list slide image slider menu stylish form table tabs text effect text scroller tooltips tree menu vertical navigation menu