Nice CSS3 and jQuery notification messages
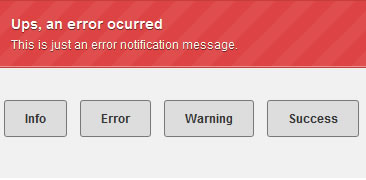
Notification messages are an important part of the user experience and you can’t afford to omit them. A notification alert message should appear every time the user perform important tasks.
The HTML
<div class="info message"> <h3>FYI, something just happened!</h3> <p>This is just an info notification message.</p> </div> <div class="error message"> <h3>Ups, an error ocurred</h3> <p>This is just an error notification message.</p> </div> <div class="warning message"> <h3>Wait, I must warn you!</h3> <p>This is just a warning notification message.</p> </div> <div class="success message"> <h3>Congrats, you did it!</h3> <p>This is just a success notification message.</p> </div>
The CSS
.message { -webkit-background-size: 40px 40px; -moz-background-size: 40px 40px; background-size: 40px 40px; background-image: -webkit-gradient(linear, left top, right bottom, color-stop(.25, rgba(255, 255, 255, .05)), color-stop(.25, transparent), color-stop(.5, transparent), color-stop(.5, rgba(255, 255, 255, .05)), color-stop(.75, rgba(255, 255, 255, .05)), color-stop(.75, transparent), to(transparent)); background-image: -webkit-linear-gradient(135deg, rgba(255, 255, 255, .05) 25%, transparent 25%, transparent 50%, rgba(255, 255, 255, .05) 50%, rgba(255, 255, 255, .05) 75%, transparent 75%, transparent); background-image: -moz-linear-gradient(135deg, rgba(255, 255, 255, .05) 25%, transparent 25%, transparent 50%, rgba(255, 255, 255, .05) 50%, rgba(255, 255, 255, .05) 75%, transparent 75%, transparent); background-image: -ms-linear-gradient(135deg, rgba(255, 255, 255, .05) 25%, transparent 25%, transparent 50%, rgba(255, 255, 255, .05) 50%, rgba(255, 255, 255, .05) 75%, transparent 75%, transparent); background-image: -o-linear-gradient(135deg, rgba(255, 255, 255, .05) 25%, transparent 25%, transparent 50%, rgba(255, 255, 255, .05) 50%, rgba(255, 255, 255, .05) 75%, transparent 75%, transparent); background-image: linear-gradient(135deg, rgba(255, 255, 255, .05) 25%, transparent 25%, transparent 50%, rgba(255, 255, 255, .05) 50%, rgba(255, 255, 255, .05) 75%, transparent 75%, transparent); -moz-box-shadow: inset 0 -1px 0 rgba(255,255,255,.4); -webkit-box-shadow: inset 0 -1px 0 rgba(255,255,255,.4); box-shadow: inset 0 -1px 0 rgba(255,255,255,.4); width: 100%; border: 1px solid; color: #fff; padding: 15px; position: fixed; _position: absolute; text-shadow: 0 1px 0 rgba(0,0,0,.5); -webkit-animation: animate-bg 5s linear infinite; -moz-animation: animate-bg 5s linear infinite; } .info { background-color: #4ea5cd; border-color: #3b8eb5; } .error { background-color: #de4343; border-color: #c43d3d; } .warning { background-color: #eaaf51; border-color: #d99a36; } .success { background-color: #61b832; border-color: #55a12c; } .message h3 { margin: 0 0 5px 0; } .message p { margin: 0; } @-webkit-keyframes animate-bg { from { background-position: 0 0; } to { background-position: -80px 0; } } @-moz-keyframes animate-bg { from { background-position: 0 0; } to { background-position: -80px 0; } }
Note the animate-bg
, which animate the background diagonal stripes. Of course, to see this effect, you should use latest Webkit browsers like Chrome/Safari or Mozilla 5+.
The notification messages will be hidden initially. For that we’ll use fixed positioning (absolute
value just for IE6 – as there is no position:fixed
support).
position: fixed; _position: absolute; /* IE6 only */
The jQuery
Define the messages types using an array:
var myMessages = ['info','warning','error','success'];
The below function hides the notification messages. Messages could have dynamic heights and for that, each message’s height is calculated in order to hide it properly.
function hideAllMessages() { var messagesHeights = new Array(); // this array will store height for each for (i=0; i<myMessages.length; i++) { messagesHeights[i] = $('.' + myMessages[i]).outerHeight(); // fill array $('.' + myMessages[i]).css('top', -messagesHeights[i]); //move element outside viewport } }
The showMessage
function is called when document ready.
function showMessage(type) { $('.'+ type +'-trigger').click(function(){ hideAllMessages(); $('.'+type).animate({top:"0"}, 500); }); }
On page load, first of all we’ll hide all the messages: hideAllMessages()
. Then, for each trigger, show the equivalent message:
$(document).ready(function(){ // Initially, hide them all hideAllMessages(); // Show message for(var i=0;i<myMessages.length;i++) { showMessage(myMessages[i]); } // When message is clicked, hide it $('.message').click(function(){ $(this).animate({top: -$(this).outerHeight()}, 500); }); });
The artilce source:http://www.red-team-design.com/cool-notification-messages-with-css3-jquery
You might also like
Tags
accordion accordion menu animation navigation animation navigation menu carousel checkbox inputs css3 css3 menu css3 navigation date picker dialog drag drop drop down menu drop down navigation menu elastic navigation form form validation gallery glide navigation horizontal navigation menu hover effect image gallery image hover image lightbox image scroller image slideshow multi-level navigation menus rating select dependent select list slide image slider menu stylish form table tabs text effect text scroller tooltips tree menu vertical navigation menu