Rotating hover Image with JQuery
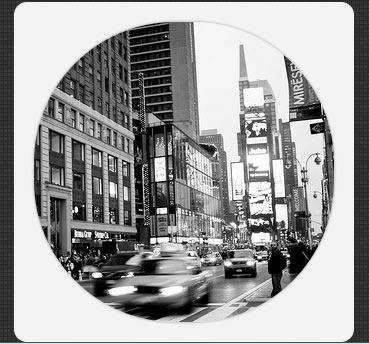
Creating a jquery plugin is easier than it sound, first time I heard about creating 3rd party plugin sounds scary, maybe need more deeper understanding about the platform, but not in jquery. Well I just feel something missing if I have been using jquery for a while not to creating a plugin.
Now just straight to the plugin code, this is the code I put on file jquery.rotate.js
(function( $ ) { $.fn.myrotate = function() { var img = this.find("img"); var imgpos = img.position(); var x0, y0; $(window).load(function() { img.removeAttr("width"); img.removeAttr("height"); x0 = imgpos.left + (img.width() / 2); y0 = imgpos.top + (img.height() / 2); }); var x, y, x1, y1, drag = 0; img.css({ "cursor": "pointer", "position": "relative" }); img.mousemove(function(e) { x1 = e.pageX; y1 = e.pageY; x = x1 - x0; y = y1 - y0; r = 360 - ((180/Math.PI) * Math.atan2(y,x)); if (drag == 1) { img.css("transform","rotate(-"+r+"deg)"); img.css("-moz-transform","rotate(-"+r+"deg)"); img.css("-webkit-transform","rotate(-"+r+"deg)"); img.css("-o-transform","rotate(-"+r+"deg)"); } }); img.mousedown(function() { if (drag == 0) { drag = 1; img.css("-webkit-box-shadow", "0 0 5px #999"); img.css("-moz-box-shadow", "0 0 5px #999"); img.css("box-shadow", "0 0 5px #999"); } else { drag = 0; img.css("-webkit-box-shadow", "0 0 2px #999"); img.css("-moz-box-shadow", "0 0 2px #999"); img.css("box-shadow", "0 0 2px #999"); } }); img.mouseleave(function() { drag = 0; }); }; })( jQuery );
The idea is similar from before, to get the image center point and get the cursor coordinate using javascript Math we can find the radian, and rotate the image using CSS. Here are a sample usage
<!DOCTYPE html> <html> <head> <title></title> <meta http-equiv="Content-Type" content="text/html; charset=UTF-8"> <script src="jquery-1.6.4.min.js"></script> <script src="jquery.rotate.js"></script> <script> $(function() { $("#content").myrotate(); $("#circle").myrotate(); }); </script> </head> <body> <div id="container"> <div id="content"> <img src="img.jpg"/> </div> <div id="circle"> <img src="img.jpg"/> </div> </div> </body> </html>
The article source:http://superdit.com/2011/12/04/jquery-plugin-for-rotating-image/
You might also like
Tags
accordion accordion menu animation navigation animation navigation menu carousel checkbox inputs css3 css3 menu css3 navigation date picker dialog drag drop drop down menu drop down navigation menu elastic navigation form form validation gallery glide navigation horizontal navigation menu hover effect image gallery image hover image lightbox image scroller image slideshow multi-level navigation menus rating select dependent select list slide image slider menu stylish form table tabs text effect text scroller tooltips tree menu vertical navigation menu