jQuery Plugin: rotate3Di - Flip HTML content in 3D
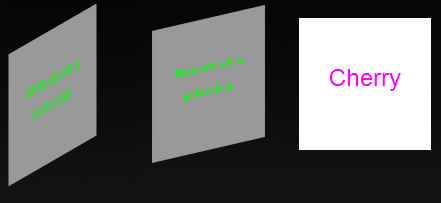
Rotate3Di is a jQuery Effect Plugin that makes it possible to do an isometric 3D flip or 3D rotation of any HTML content. It also enables custom 3D rotation animations. CSS Transforms are used to create this visual "3D" isometric effect. Supported browsers are WebKit, Safari, Chrome, and Firefox 3.5. The plugin's functionality includes: setting or animating HTML content to an arbitrary isometric rotation angle, as well as flipping, unflipping, or toggling the flip state of an object.
Getting Started
In order to use the rotate3Di jQuery plugin, your web page will need to include jQuery v1.2.6 or newer,, the jQuery CSS Transform patch, and the rotate3Di plugin itself
<script type="text/javascript" src="rotate3Di-0.9/jquery-1.3.2.js"></script> <script type="text/javascript" src="rotate3Di-0.9/jquery-css-transform.js"></script> <script type="text/javascript" src="rotate3Di-0.9/rotate3Di-0.9.js"></script>
Rotate3Di can then be used like other jQuery Effects:
$('#rot-ex').rotate3Di(180, 3000);
Usage
Statically Setting a Rotation Angle
Rotate3Di can be used to statically set the isometric rotation angle of an object.
Code:
$('#ex1').rotate3Di(30);
Animating to a Rotation Angle
Rotate3Di can animate an object to any angle of rotation about the isometric Y axis. Click the image to see the rotation animated. Note that once the animation has finished, the object will be at a 180 degree angle to the Y axis already, thus repeat clicks will have no effect.
Code:
$('#ex2').click(function () {$(this).rotate3Di(180, 1000);});
Animating Relative Degrees of Rotation
Rotate3Di can animate the rotation of an object through any number of degrees of rotation about the isometric Y axis. Click the image to see the animated rotation, this time by -180 degrees (thus, counterclockwise rotation). Click the image additional times to rotate by the same amount again and again.
Code:
$('#ex3').click(function () {$(this).rotate3Di('-=180', 1000);});
Flip, Unflip, and Toggle Shortcuts
With rotate3Di, it is possible to flip, unflip, or toggle the flip state of an object without specifying numerical degree values.
Code:
// Two things to note here: // 1. We use .stop() to prevent the "buildup" of animations // 2. We capture the hover event on a different element than the one we // rotate so that the hover-out isn't triggered if the rotation of the // element moves it out from under the user's mouse pointer. $('#ex4').hover( function () {$(this).find('p').stop().rotate3Di('flip', 500);}, function () {$(this).find('p').stop().rotate3Di('unflip', 500);} ); $('#ex5').click(function () {$(this).rotate3Di('toggle', 1000);}); $('#ex6').click(function () { $(this).rotate3Di('toggle', 1000, {direction: 'clockwise'}); });
Using Callback Functions for Side Change and Animation Completion
Using the callback function options available with rotate3Di, you can take action as your object reveals its front and back sides during rotation, as well as take action when your animation has completed. Click this example to animate it.
Code:
function mySideChange(front) { if (front) { $(this).css('background', '#f0f'); } else { $(this).css('background', '#0f0'); } } function myComplete() { $('#ex7').css('backgroundColor', '#f00'); } $('#ex7').click(function () { $(this).rotate3Di( '360', 3000, { sideChange: mySideChange, complete: myComplete } ); });
You might also like
Tags
accordion accordion menu animation navigation animation navigation menu carousel checkbox inputs css3 css3 menu css3 navigation date picker dialog drag drop drop down menu drop down navigation menu elastic navigation form form validation gallery glide navigation horizontal navigation menu hover effect image gallery image hover image lightbox image scroller image slideshow multi-level navigation menus rating select dependent select list slide image slider menu stylish form table tabs text effect text scroller tooltips tree menu vertical navigation menu