Fast and Simple Toggle View Content with jQuery




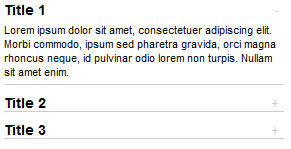
This script is fairly straight forward. What it does is using the UL list and allow user to toggle to display the content of LI items. This is a really useful user interface feature that it helps designers to save space and build a less complicated user interface.
1. HTML
It consists of UL list and the rest is quite self-explanatory.
<ul id="toggle-view"> <li> <h3>Title 1</h3> <span>+</span> <p>Lorem ipsum dolor sit amet, consectetuer adipiscing elit. Morbi commodo, ipsum sed pharetra gravida, orci magna rhoncus neque, id pulvinar odio lorem non turpis. Nullam sit amet enim.</p> </li> <li> <h3>Title 2</h3> <span>+</span> <p>Lorem ipsum dolor sit amet, consectetuer adipiscing elit. Morbi commodo, ipsum sed pharetra gravida, orci magna rhoncus neque, id pulvinar odio lorem non turpis. Nullam sit amet enim.</p> </li> <li> <h3>Title 3</h3> <span>+</span> <p>Lorem ipsum dolor sit amet, consectetuer adipiscing elit. Morbi commodo, ipsum sed pharetra gravida, orci magna rhoncus neque, id pulvinar odio lorem non turpis. Nullam sit amet enim.</p> </li> </ul>
2. CSS
I used the minimum CSS code to style this tutorial. The most important thing is, we have to make sure the P is hidden by default.
#toggle-view { list-style:none; font-family:arial; font-size:11px; margin:0; padding:0; width:300px; } #toggle-view li { margin:10px; border-bottom:1px solid #ccc; position:relative; cursor:pointer; } #toggle-view h3 { margin:0; font-size:14px; } #toggle-view span { position:absolute; right:5px; top:0; color:#ccc; font-size:13px; } #toggle-view p { margin:5px 0; display:none; }
3. Javascript
This would be one of my shortest jQuery code! We attach click event to the LI item and everytime a user click on the item check if P tag is visible. If it's hidden, show it otherwise hide it. Also, it will change the html value for the SPAN to either plus or negative sign.
$(document).ready(function () { $('#toggle-view li').click(function () { var text = $(this).children('p'); if (text.is(':hidden')) { text.slideDown('200'); $(this).children('span').html('-'); } else { text.slideUp('200'); $(this).children('span').html('+'); } }); });
You might also like
Tags
accordion accordion menu animation navigation animation navigation menu carousel checkbox inputs css3 css3 menu css3 navigation date picker dialog drag drop drop down menu drop down navigation menu elastic navigation form form validation gallery glide navigation horizontal navigation menu hover effect image gallery image hover image lightbox image scroller image slideshow multi-level navigation menus rating select dependent select list slide image slider menu stylish form table tabs text effect text scroller tooltips tree menu vertical navigation menu