iPhone Style Radio and Checkbox Switches using jQuery and CSS




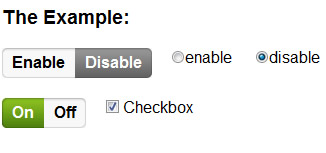
Prerequisites
You need just two things for this to work correctly: JQuery 1.3.2+ and the switch.gif image file used for the backgrounds (both included in the zip file). After you have those two files, follow the steps below:
Step 1 The HTML
<p class="field switch"> <input type="radio" id="radio1" name="field" checked /> <input type="radio" id="radio2" name="field" /> <label for="radio1" class="cb-enable selected"><span>Enable</span></label> <label for="radio2" class="cb-disable"><span>Disable</span></label> </p> <p class="field switch"> <label class="cb-enable"><span>On</span></label> <label class="cb-disable selected"><span>Off</span></label> <input type="checkbox" id="checkbox" class="checkbox" name="field2" /> </p>
Step 2 The Javascript
$(document).ready( function(){ $(".cb-enable").click(function(){ var parent = $(this).parents('.switch'); $('.cb-disable',parent).removeClass('selected'); $(this).addClass('selected'); $('.checkbox',parent).attr('checked', true); }); $(".cb-disable").click(function(){ var parent = $(this).parents('.switch'); $('.cb-enable',parent).removeClass('selected'); $(this).addClass('selected'); $('.checkbox',parent).attr('checked', false); }); });
Step 3 The CSS
.cb-enable, .cb-disable, .cb-enable span, .cb-disable span { background: url(switch.gif) repeat-x; display: block; float: left; } .cb-enable span, .cb-disable span { line-height: 30px; display: block; background-repeat: no-repeat; font-weight: bold; } .cb-enable span { background-position: left -90px; padding: 0 10px; } .cb-disable span { background-position: right -180px;padding: 0 10px; } .cb-disable.selected { background-position: 0 -30px; } .cb-disable.selected span { background-position: right -210px; color: #fff; } .cb-enable.selected { background-position: 0 -60px; } .cb-enable.selected span { background-position: left -150px; color: #fff; } .switch label { cursor: pointer; } .switch input { display: none; }
Alternatively, you could use a single hidden input field and change the value of it using JQuery. I used this method mainly to illustrate the effect works, as you can actually see the form values changing (to make sure it works).
I know it doesn’t have the fancy animation effects of the alternatives but give it a shot and let me know what you guys think!
You might also like
Tags
accordion accordion menu animation navigation animation navigation menu carousel checkbox inputs css3 css3 menu css3 navigation date picker dialog drag drop drop down menu drop down navigation menu elastic navigation form form validation gallery glide navigation horizontal navigation menu hover effect image gallery image hover image lightbox image scroller image slideshow multi-level navigation menus rating select dependent select list slide image slider menu stylish form table tabs text effect text scroller tooltips tree menu vertical navigation menu